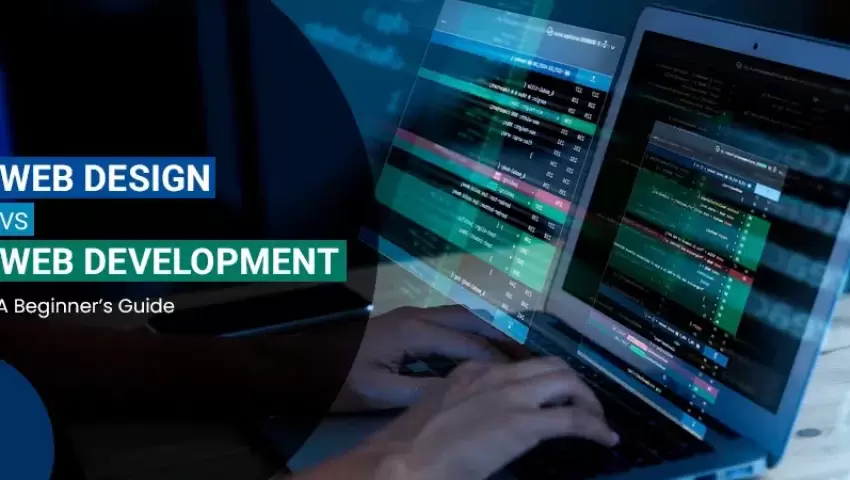
A Beginner’s Guide to Web Development
Understand the DOM and how it impacts dynamic web development. Learn how to manipulate elements, handle events, and improve website interactivity using JavaScript.
Understanding the DOM: A Beginner’s Guide to Web Development
The Document Object Model (DOM) is an essential concept for web developers. It provides a structured representation of a web page that allows developers to access and manipulate content, structure, and style dynamically. Whether you’re building static websites or dynamic web apps, understanding how the DOM works is critical to building interactive, high-performing sites.
In this blog, we’ll break down what the DOM is, how it functions, and how you can use it effectively in your web development projects. By the end, you’ll have a solid understanding of the DOM and how it relates to web page rendering, JavaScript, and dynamic content manipulation.
What is the DOM?
At its core, the DOM is a programming interface for web documents. It represents the structure of a web page as a tree-like structure where each node corresponds to an element on the page. In simpler terms, the DOM is an in-memory representation of the HTML or XML document that can be modified via scripting languages like JavaScript.
When a browser loads a webpage, it parses the HTML content and builds the DOM tree. Each HTML element becomes an object in the DOM, which JavaScript can then manipulate, allowing developers to dynamically update the page's content, structure, and styles without needing to reload the entire page.
How the DOM Works
The DOM provides a way for JavaScript to interact with the web page's HTML and CSS. Here’s a breakdown of how it works:
- HTML to DOM Conversion When a browser loads an HTML document, it parses the HTML code and creates a DOM tree. For example, an HTML
<div>
element becomes adiv
node in the DOM. The browser uses this tree structure to render the page content. - DOM Tree Structure The DOM organizes HTML elements in a hierarchical structure. The root node of the DOM is typically the document object, and the child nodes represent various HTML elements like
<body>
,<div>
,<p>
,<h1>
, etc. Each element can have attributes (likeclass
orid
) and text content. - Interacting with the DOM JavaScript can interact with the DOM by selecting elements and manipulating their properties, attributes, or content. For example, you can change the text inside a
<p>
tag, update the style of an element, or add new elements dynamically to the page. - DOM Events The DOM also handles events such as clicks, mouse movements, keypresses, and form submissions. JavaScript can listen for these events and execute specific actions in response. This allows developers to create interactive web pages and apps that respond to user input in real time.
DOM Manipulation with JavaScript
Manipulating the DOM is an essential skill for developers, as it allows you to modify a page’s structure and content dynamically. Here are some common methods for manipulating the DOM with JavaScript:
- Selecting Elements To manipulate an element, you first need to select it using JavaScript. The most common methods for selecting elements are:
document.getElementById()
: Selects an element by its uniqueid
attribute.document.getElementsByClassName()
: Selects all elements with a specific class.document.querySelector()
: Selects the first element that matches a CSS selector.document.querySelectorAll()
: Selects all elements that match a CSS selector.
- Modifying Content Once you’ve selected an element, you can modify its content using the following properties:
.innerHTML
: Sets or gets the HTML content inside an element..textContent
: Sets or gets the plain text content inside an element..value
: Used for form elements like<input>
and<textarea>
to modify their values.
- Changing Attributes You can change an element’s attributes, such as
src
,href
,alt
, orstyle
, using JavaScript. The following methods allow you to modify attributes:.setAttribute()
: Sets a new value for an attribute..getAttribute()
: Retrieves the value of an attribute..removeAttribute()
: Removes an attribute from an element.
- Manipulating Styles JavaScript can modify the inline styles of elements. For example:
.style
: Allows you to directly change the CSS properties of an element, such aselement.style.backgroundColor = 'blue';
.
- Adding and Removing Elements The DOM also allows you to add, remove, or clone elements dynamically:
document.createElement()
: Creates a new HTML element.parentNode.appendChild()
: Appends a new element as a child to a parent.parentNode.removeChild()
: Removes an element from the DOM.
Understanding the DOM Tree Structure
The DOM tree structure is hierarchical, with the root node being the document
. Here’s an example of a basic DOM structure for a simple HTML document:
The DOM representation of the above HTML document would look like this:
As you can see, the DOM is organized as a tree, where each HTML tag becomes a node, and the parent-child relationships between elements are preserved.
Common Issues in DOM Manipulation
While DOM manipulation is a powerful tool, it’s important to be aware of common issues that may arise:
- Performance Considerations Frequent DOM manipulation can lead to performance issues, especially on large websites with complex structures. To optimize performance, minimize direct DOM manipulation and consider using JavaScript frameworks or libraries like React or Vue, which efficiently handle DOM updates.
- Cross-browser Compatibility Different browsers may implement the DOM in slightly different ways. Make sure to test your web apps on multiple browsers to ensure consistent behavior. Tools like
window.console
can help debug and inspect the DOM across browsers. - DOM Manipulation in Real-Time Sometimes, JavaScript might manipulate the DOM while the page is still loading, leading to issues like "flash of unstyled content" (FOUC). To avoid this, you can use the
DOMContentLoaded
event to delay DOM manipulation until the page is fully loaded.
Best Practices for DOM Manipulation
- Minimize Direct DOM Access Direct manipulation of the DOM can be slow, so try to minimize unnecessary DOM reads and writes. Use JavaScript frameworks like React or Vue that optimize DOM interactions for you.
- Use Event Delegation Instead of attaching event listeners to each individual element, use event delegation by attaching a single listener to a parent element. This approach improves performance, especially in dynamic applications.
- Cache DOM References If you need to interact with the same element multiple times, consider caching its reference in a variable to avoid querying the DOM repeatedly, which can improve performance.
- Use CSS for Animations When creating animations or transitions, use CSS instead of JavaScript whenever possible. CSS animations are generally faster and more efficient.
Mastering the DOM for Dynamic Web Development
Understanding the DOM is fundamental to modern web development. It empowers developers to create dynamic, interactive web applications by enabling real-time content manipulation, event handling, and more. By mastering DOM manipulation, you’ll be able to create websites and apps that are not only engaging but also high-performing and user-friendly.
Whether you’re working with vanilla JavaScript or using libraries and frameworks, a strong grasp of the DOM will allow you to unlock powerful capabilities in web development.
Zurihub Admin
Leave a comment
Your email address will not be published. Required fields are marked *